Ruby Openssl Generate Rsa Key
OpenSSL provides SSL, TLS and generalpurpose cryptography. It wraps the OpenSSL library.
Office 2007 enterprise activation key generator. Microsoft office 2016 activation key generator. OpenSSL comes bundled with the Standard Library of Ruby.
- Version of OpenSSL the ruby OpenSSL extension is running with. OPENSSLVERSION Version of OpenSSL the ruby OpenSSL extension was built with. OPENSSLVERSIONNUMBER Version number of OpenSSL the ruby OpenSSL extension was built with (base 16) VERSION OpenSSL ruby extension version. Public Class Methods.
- How do I create an OpenSSL::PKey object initialized with the following public key string? End goal is to use the object to decode a JWT token using ruby-jwt. I've tried the following: publickey.
- OpenSSL provides SSL, TLS and general purpose cryptography.It wraps the OpenSSL library. Examples ¶ ↑. All examples assume you have loaded OpenSSL with. Require 'openssl'. These examples build atop each other.
Generates or loads an RSA keypair. If an integer keysize is given it represents the desired key size. Keys less than 1024 bits should be considered insecure. A key can instead be loaded from an encodedkey which must be PEM or DER encoded. A passphrase can be used to decrypt the key. If none is given OpenSSL will prompt for the pass phrase. Examples ¶ ↑. Generate a 2048 bit RSA Key. You can generate a public and private RSA key pair like this: openssl genrsa -des3 -out private.pem 2048. That generates a 2048-bit RSA key pair, encrypts them with a password you provide and writes them to a file. You need to next extract the public key file.
This means the OpenSSL extension is compiled with Ruby and packaged onbuild. During compile time, Ruby will need to link against the OpenSSLlibrary on your system. However, you cannot use openssl provided by Appleto build standard library openssl.
If you use OSX, you should install another openssl and run “`./configure–with-openssl-dir=/path/to/another-openssl“`. For Homebrew user, run `brewinstall openssl` and then “`./configure –with-openssl-dir=`brew –prefixopenssl` “`.
All examples assume you have loaded OpenSSL with:
These examples build atop each other. For example the key created in thenext is used in throughout these examples.
Keys
Creating a Key
This example creates a 2048 bit RSA keypair and writes it to the currentdirectory.
Exporting a Key
Keys saved to disk without encryption are not secure as anyone who getsahold of the key may use it unless it is encrypted. In order to securelyexport a key you may export it with a pass phrase.
OpenSSL::Cipher.ciphersreturns a list of available ciphers.
Loading a Key
A key can also be loaded from a file.
or
Loading an Encrypted Key
OpenSSL will prompt you for your pass phrase when loading an encrypted key.If you will not be able to type in the pass phrase you may provide it whenloading the key:
RSA Encryption
RSA provides encryption and decryption using the public and private keys.You can use a variety of padding methods depending upon the intended use ofencrypted data.
Encryption & Decryption
Asymmetric public/private key encryption is slow and victim to attack incases where it is used without padding or directly to encrypt larger chunksof data. Typical use cases for RSA encryption involve “wrapping” asymmetric key with the public key of the recipient who would “unwrap” thatsymmetric key again using their private key. The following illustrates asimplified example of such a key transport scheme. It shouldn’t be used inpractice, though, standardized protocols should always be preferred.
A symmetric key encrypted with the public key can only be decrypted withthe corresponding private key of the recipient.
By default PKCS#1 padding will be used, but it is also possible to useother forms of padding, see PKey::RSAfor further details.
Signatures
Using “private_encrypt” to encrypt some data with the private key isequivalent to applying a digital signature to the data. A verifying partymay validate the signature by comparing the result of decrypting thesignature with “public_decrypt” to the original data. However, OpenSSL::PKey already has methods “sign” and“verify” that handle digital signatures in a standardized way -“private_encrypt” and “public_decrypt” shouldn’t be used in practice.
To sign a document, a cryptographically secure hash of the document iscomputed first, which is then signed using the private key.
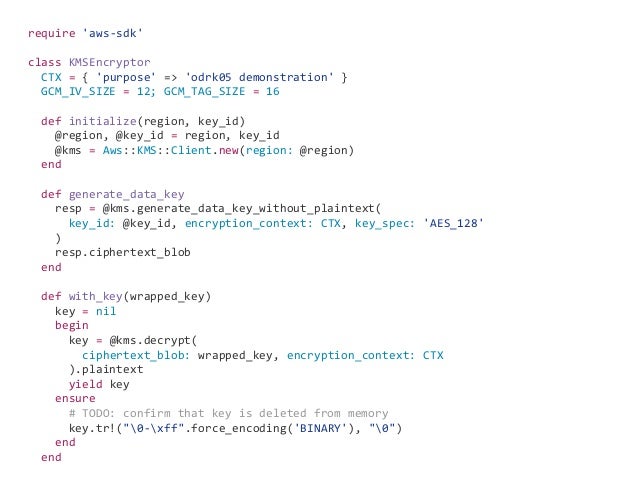
To validate the signature, again a hash of the document is computed and thesignature is decrypted using the public key. The result is then compared tothe hash just computed, if they are equal the signature was valid.
PBKDF2 Password-based Encryption
If supported by the underlying OpenSSL version used, Password-basedEncryption should use the features of PKCS5. If not supported or if required bylegacy applications, the older, less secure methods specified in RFC 2898are also supported (see below).
PKCS5 supports PBKDF2 as it was specifiedin PKCS#5 v2.0.It still uses a password, a salt, and additionally a number of iterationsthat will slow the key derivation process down. The slower this is, themore work it requires being able to brute-force the resulting key.
Encryption
The strategy is to first instantiate a Cipher for encryption, and then to generatea random IV plus a key derived from the password using PBKDF2. PKCS #5 v2.0recommends at least 8 bytes for the salt, the number of iterations largelydepends on the hardware being used.
Decryption
Use the same steps as before to derive the symmetric AES key, this timesetting the Cipher up for decryption.
PKCS #5 Password-based Encryption
PKCS #5 is a password-based encryption standard documented at RFC2898. It allows a shortpassword or passphrase to be used to create a secure encryption key. Ifpossible, PBKDF2 as described above should be used if the circumstancesallow it.
PKCS #5 uses a Cipher, a pass phrase anda salt to generate an encryption key.
Encryption
First set up the cipher for encryption
Ruby Openssl Generate Rsa Key On Cisco Router
Then pass the data you want to encrypt through
Decryption
Use a new Cipher instance set up fordecryption
Then pass the data you want to decrypt through
X509 Certificates
Creating a Certificate
This example creates a self-signed certificate using an RSA key and a SHA1signature.
Ruby Openssl Generate Rsa Key Windows
Certificate Extensions
You can add extensions to the certificate withOpenSSL::SSL::ExtensionFactory to indicate the purpose of the certificate.
The list of supported extensions (and in some cases their possible values)can be derived from the “objects.h” file in the OpenSSL source code.
Signing a Certificate
To sign a certificate set the issuer and use OpenSSL::X509::Certificate#signwith a digest algorithm. This creates a self-signed cert because we’reusing the same name and key to sign the certificate as was used to createthe certificate.
Loading a Certificate
Like a key, a cert can also be loaded from a file.
Verifying a Certificate
Certificate#verify will return true when a certificate was signed with thegiven public key.
Openssl Create Private Key
Certificate Authority
A certificate authority (CA) is a trusted third party that allows you toverify the ownership of unknown certificates. The CA issues key signaturesthat indicate it trusts the user of that key. A user encountering the keycan verify the signature by using the CA’s public key.
CA Key
CA keys are valuable, so we encrypt and save it to disk and make sure it isnot readable by other users.
CA Certificate
A CA certificate is created the same way we created a certificate above,but with different extensions.
This extension indicates the CA’s key may be used as a CA.
This extension indicates the CA’s key may be used to verify signatures onboth certificates and certificate revocations.
Root CA certificates are self-signed.
The CA certificate is saved to disk so it may be distributed to all theusers of the keys this CA will sign.
Certificate Signing Request
The CA signs keys through a Certificate Signing Request (CSR). The CSRcontains the information necessary to identify the key.
Ruby Openssl Generate Rsa Key Pair
A CSR is saved to disk and sent to the CA for signing.
Creating a Certificate from a CSR
Upon receiving a CSR the CA will verify it before signing it. A minimalverification would be to check the CSR’s signature.
After verification a certificate is created, marked for various usages,signed with the CA key and returned to the requester.
SSL and TLS Connections
Using our created key and certificate we can create an SSL or TLS connection. An SSLContext is used toset up an SSL session.
SSL Server
An SSL server requires the certificate andprivate key to communicate securely with its clients:
Then create an SSLServer with a TCP server socket and the context. Use theSSLServer like an ordinary TCP server.
SSL client
An SSL client is created with a TCP socketand the context. SSLSocket#connect must be called to initiate the SSL handshake and start encryption. A key andcertificate are not required for the client socket.
Peer Verification
An unverified SSL connection does notprovide much security. For enhanced security the client or server canverify the certificate of its peer.
The client can be modified to verify the server’s certificate against thecertificate authority’s certificate:
If the server certificate is invalid or context.ca_file
is notset when verifying peers an OpenSSL::SSL::SSLError will be raised.